AI Tutorials
How to Create Your First AI-Powered Chatbot Using Python and OpenAI: A Beginner’s Guide
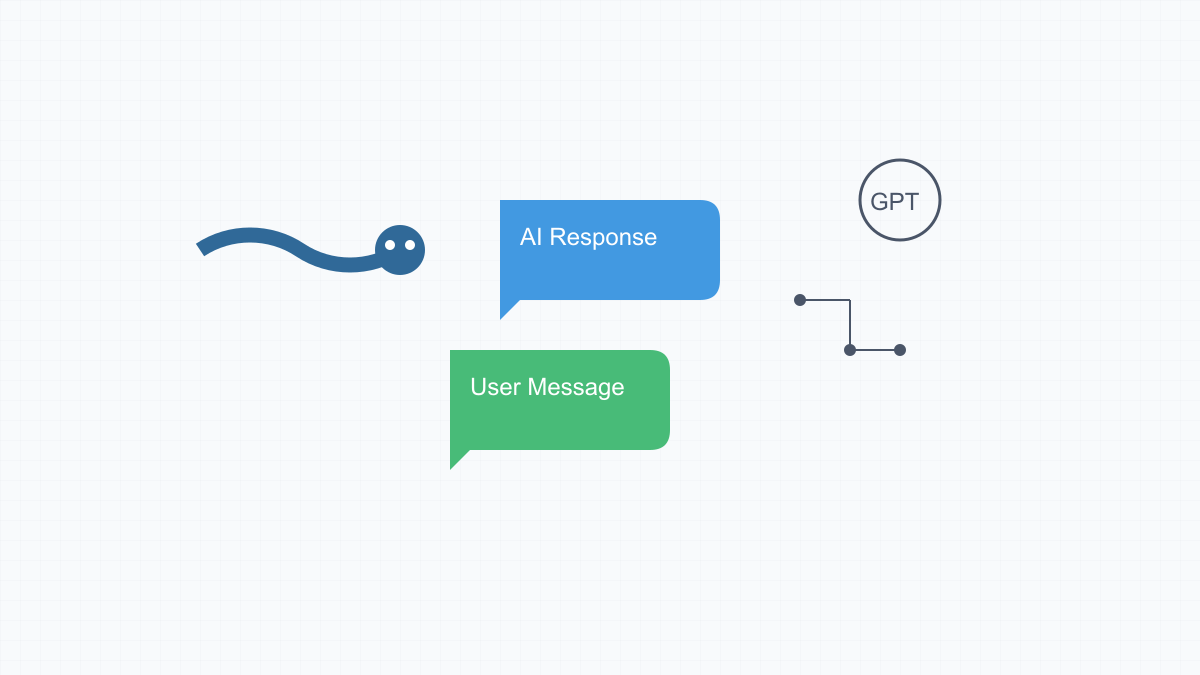
In today’s rapidly evolving tech landscape, AI chatbots have become increasingly essential tools. Although creating one might seem challenging at first, modern tools and APIs have made this process remarkably accessible. Throughout this comprehensive guide, we’ll explore how to build a functional chatbot using Python and OpenAI’s powerful language models. Furthermore, regardless of whether you’re a beginner programmer or an experienced developer venturing into AI, this tutorial will provide you with a solid foundation in chatbot development.
Prerequisites
Before we begin our journey into chatbot development, it’s essential to ensure that you have all the necessary tools and knowledge. Therefore, you’ll need:
- Basic knowledge of Python programming
- Python 3.7 or newer installed on your computer
- A text editor or IDE (such as VS Code or PyCharm)
- An OpenAI API key (which we’ll help you obtain)
- Basic familiarity with command-line operations
Getting Started with OpenAI API
Setting Up Your Development Environment
To begin with, we’ll prepare your workspace. Initially, open your terminal and create a new project directory:ment. Open your terminal and create a new project directory:
mkdir ai-chatbot
cd ai-chatbot
python -m venv venv
Subsequently, you’ll need to activate your virtual environment:
For Windows users:
venv\Scripts\activate
Alternatively, for macOS/Linux users:
source venv/bin/activate
Installing Required Dependencies
Once your environment is set up, we can proceed with installing the necessary packages. Therefore, run:
pip install openai python-dotenv requests
Getting Your OpenAI API Key
In order to access OpenAI’s API, follow these sequential steps:
- First and foremost, visit OpenAI’s website (https://platform.openai.com/signup)
- Subsequently, create an account or sign in
- After that, navigate to the API section
- Then, generate a new API key
- Finally, store your API key in a secure location
Building the Chatbot
Setting Up the Project Structure
To ensure proper organization, first create these essential files in your project directory:
ai-chatbot/
├── .env
├── config.py
├── chatbot.py
└── requirements.txt
Configuring Environment Variables
As a first step, create a .env
file to securely store your API key:
OPENAI_API_KEY=your_api_key_here
Creating the Configuration File
Moving forward, in your config.py
, add the following configuration settings:
import os
from dotenv import load_dotenv
load_dotenv()
OPENAI_API_KEY = os.getenv('OPENAI_API_KEY')
MODEL_NAME = "gpt-3.5-turbo"
MAX_TOKENS = 150
TEMPERATURE = 0.7
Implementing the Core Chatbot Functionality
Now, let’s create the main chatbot implementation in chatbot.py
:
import openai
from config import OPENAI_API_KEY, MODEL_NAME, MAX_TOKENS, TEMPERATURE
class AIChatbot:
def __init__(self):
openai.api_key = OPENAI_API_KEY
self.conversation_history = []
def generate_response(self, user_input):
try:
# Add user input to conversation history
self.conversation_history.append({"role": "user", "content": user_input})
# Generate response using OpenAI API
response = openai.ChatCompletion.create(
model=MODEL_NAME,
messages=self.conversation_history,
max_tokens=MAX_TOKENS,
temperature=TEMPERATURE
)
# Extract and store assistant's response
assistant_response = response.choices[0].message['content']
self.conversation_history.append({"role": "assistant", "content": assistant_response})
return assistant_response
except Exception as e:
return f"An error occurred: {str(e)}"
def clear_history(self):
self.conversation_history = []
Adding Advanced Features
Implementing Context Management
To enhance our chatbot, let’s add context management capabilities:
def manage_context(self, max_history=10):
if len(self.conversation_history) > max_history * 2:
# Keep the most recent conversations
self.conversation_history = self.conversation_history[-max_history*2:]
Adding Error Handling
Furthermore, let’s implement robust error handling:
def handle_errors(func):
def wrapper(*args, **kwargs):
try:
return func(*args, **kwargs)
except openai.error.RateLimitError:
return "Rate limit exceeded. Please wait a moment before trying again."
except openai.error.AuthenticationError:
return "Authentication error. Please check your API key."
except openai.error.APIError:
return "OpenAI API is currently unavailable. Please try again later."
except Exception as e:
return f"An unexpected error occurred: {str(e)}"
return wrapper
Creating a User Interface
Command-Line Interface
Additionally, let’s add a simple command-line interface for testing:
def main():
chatbot = AIChatbot()
print("AI Chatbot initialized. Type 'quit' to exit.")
while True:
user_input = input("\nYou: ")
if user_input.lower() == 'quit':
break
response = chatbot.generate_response(user_input)
print(f"\nChatbot: {response}")
if __name__ == "__main__":
main()
Best Practices and Optimization
Performance Optimization
First, let’s implement batch processing for multiple messages:
def process_batch(self, messages, batch_size=5):
responses = []
for i in range(0, len(messages), batch_size):
batch = messages[i:i + batch_size]
batch_responses = [self.generate_response(msg) for msg in batch]
responses.extend(batch_responses)
return responses
Security Considerations
Moreover, let’s implement these important security measures:
- Input validation
- Rate limiting
- Content filtering
def validate_input(self, user_input):
# Basic input validation
if not user_input or len(user_input) > 1000:
return False
return True
def filter_content(self, text):
# Basic content filtering
prohibited_words = ['harmful_word1', 'harmful_word2']
return not any(word in text.lower() for word in prohibited_words)
Testing Your Chatbot
Unit Testing
Now, create a test_chatbot.py
file:
import unittest
from chatbot import AIChatbot
class TestChatbot(unittest.TestCase):
def setUp(self):
self.chatbot = AIChatbot()
def test_response_generation(self):
response = self.chatbot.generate_response("Hello!")
self.assertIsNotNone(response)
self.assertIsInstance(response, str)
def test_input_validation(self):
self.assertTrue(self.chatbot.validate_input("Hello"))
self.assertFalse(self.chatbot.validate_input(""))
Deploying Your Chatbot
Local Deployment
To deploy locally, follow these steps:
- First, ensure all dependencies are in
requirements.txt
:
pip freeze > requirements.txt
- Then, create a simple deployment script:
import subprocess
import sys
def deploy_local():
subprocess.check_call([sys.executable, "-m", "pip", "install", "-r", "requirements.txt"])
subprocess.check_call([sys.executable, "chatbot.py"])
Troubleshooting Common Issues
Common Problems and Solutions
Here are the most common issues you might encounter:
- API Key Issues
- First, verify key validity
- Then, check environment variable loading
- Finally, ensure proper key permissions
- Rate Limiting
- Initially, implement exponential backoff
- Subsequently, use caching for frequent requests
- Additionally, monitor API usage
- Context Management
- First, perform regular cleanup of conversation history
- Then, implement proper session management
- Finally, handle timeout scenarios
Conclusion
In conclusion, you have successfully created a fully functional AI-powered chatbot using Python and OpenAI’s API. As a result of following this tutorial, you now have a solid foundation for further development. Additionally, you can enhance this foundation with various features such as:
- Natural Language Processing improvements
- Multiple personality modes
- Integration with other platforms
- Database storage for conversations
- Web interface implementation
Most importantly, remember to regularly update your dependencies and stay informed about OpenAI’s API changes and best practices.
Additional Resources
To further enhance your knowledge and skills, consider exploring these valuable resources:
- OpenAI API Documentation
- Python Official Documentation
- Real Python Tutorials
- Machine Learning Mastery
Next Steps
Finally, to continue improving your chatbot, consider following this recommended sequence:
- First and foremost, experiment with different model parameters
- Subsequently, add more sophisticated error handling
- Following that, implement additional features like voice integration
- Then, create a web interface using Flask or Django
- Ultimately, deploy your chatbot to a cloud platform
In the final analysis, remember to maintain your code quality, consistently update your dependencies, and diligently follow security best practices as you continue developing your AI chatbot. Above all, keep experimenting and learning from each iteration of your development process.t.
You may like
-
AI Language Models Display Human-like Cognitive Limitations, New Study Reveals
-
Workday Layoffs Accelerate AI Transformation as Tech Giant Shifts Focus
-
AI Analysis Software Being Deployed to Review Federal Agency Data
-
US Senators Push for Stricter Chip Controls Amid Chinese AI Advances
-
Google Removes AI Weapons Ban: Major Shift in Ethical Guidelines
-
AI Development Tools Every Programmer Should Know in 2025